Busses
Busses are a collection of analog or logic nets of the same communication protocol. This enables the ability to decode the information being sent over a particular bus in order to help debug what is being sent and received.
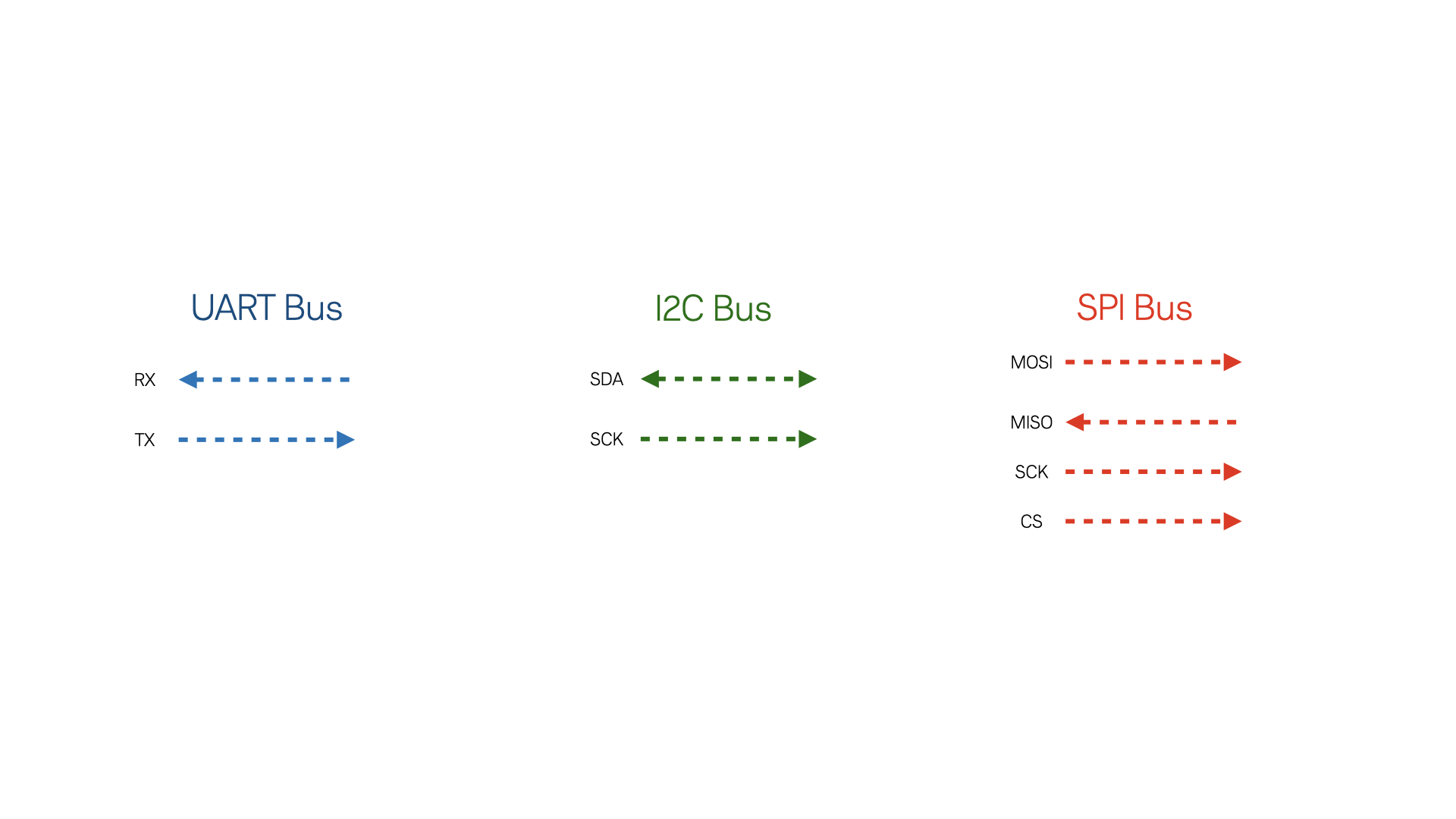
Example Communication Busses
Creating and Enabling A Bus
Before creating a bus, the component signals of the bus need to be instantiated. Then those are used as parameters for creating the given bus.
example_create_bus.py
from lager import Net, NetType, UART
tx = Net.get('UART.TX',
type=NetType.Logic,
setup_function=setup_nets,
teardown_function=teardown_nets)
rx = Net.get('UART.RX',
type=NetType.Logic,
setup_function=setup_nets,
teardown_function=teardown_nets)
uart = UART(tx=tx,rx=rx)
uart.enable()
Example of Creating A Bus
~ lager bus uart --source-tx UART.TX --source-rx UART.RX --dut 1
Data Formatting and Presentation
Regardless of bus type, decoded data can be presented in either ASCII, HEX, Decimal, or Binary format. The decoded data can be presented to you via the webapp the terminal or a python script.
format_data_ascii.py
from lager import Net, NetType, UART
tx = Net.get('UART.TX',
type=NetType.Logic,
setup_function=setup_nets,
teardown_function=teardown_nets)
rx = Net.get('UART.RX',
type=NetType.Logic,
setup_function=setup_nets,
teardown_function=teardown_nets)
uart = UART(tx=tx,rx=rx)
uart.format_ascii()
Format Data As ASCII
~ lager bus format ascii --dut 1
retreive_bus_data.py
from lager import Net, NetType, UART
tx = Net.get('UART.TX',
type=NetType.Logic,
setup_function=setup_nets,
teardown_function=teardown_nets)
rx = Net.get('UART.RX',
type=NetType.Logic,
setup_function=setup_nets,
teardown_function=teardown_nets)
uart = UART(tx=tx,rx=rx)
print(uart.bus_data())
#save data
uart.save_bus_data("path/to/file.csv")